Basic Python Programs for Beginners – Part 1
Watch This Video session for How to Run your First Python Program
# Sum of two numbers program on python
a=int(input("Enter Num1:")) b=int(input("Enter Num2:")) c=a+b print "sum of {0} and {1} is {2}" .format(a,b,c) c=a-b print "subtraction of {0} from {1} is {2}".format(a,b,c) c=a*b print "muliplication of {0} and {1} is {2}".format(a,b,c) c=a/b print "division of {0} by {1} is {2}".format(a,b,c)
Output:
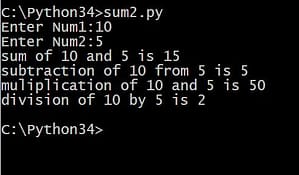
# Program to print simple and compound interest
p=float(input("Enter Principle amount:")) t=float(input("Time Duration:")) r=float(input("Enter Rate of int:")) si=(p*t*r)/100; print "simple int = ", si ci=p*(1+r/100)*r print "compound int = ", ci
Output:

# Find area of circle
r=float(input("Enter radius:")) PI=3.142 area=PI*(r*r); print "Area is= ", area
Output:

# if and else statements
amount = int(input("Enter amount: ")) if amount<5000: discount = amount*0.05 print ("Discount",discount) else: discount = amount*0.20 print "Discount",discount print "Net payable:",amount-discount
Output:

#Program to check, entered number is Even or Odd
x=int(input("Enter any number:")) if (x%2==0): print("Even"); else: print("Odd");
Output:

# largest of three numbers using python
a=int(input("Enter num1:")) b=int(input("Enter num2:")) c=int(input("Enter num3:")) if ((a>b) and (a>c)): print("a is large") elif ((b>a) and (b>c)): print("b is large") else: print("c is large")
Output:
